Data Types
Articles on Elixir
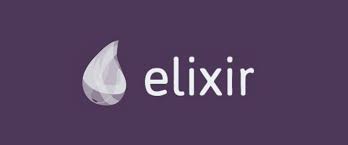
August 22, 2023
Overview →
Elixir uses Data Types
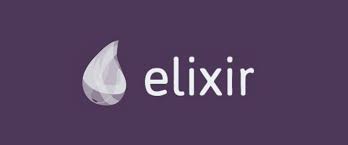
March 15, 2022
List and Map Function: Enums →
Here are a few Enum functions for Lists and Maps
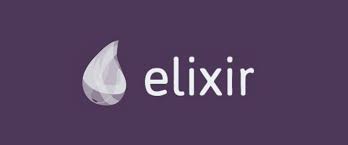
August 22, 2023
Lists →
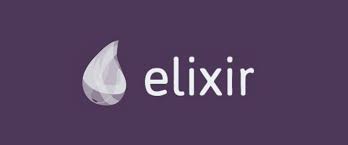
September 22, 2023
Map Functions →
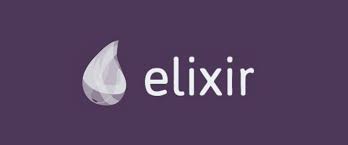
September 22, 2023
Maps →
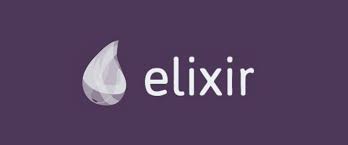
August 22, 2023
Strings →
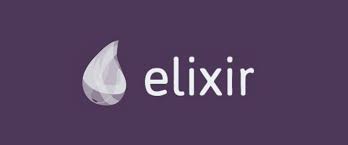
August 22, 2023
Structs →
Structs